Kirbyfree flash games. A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
This blog post is a coding and system design interview cookbook/cheatsheet compiled by me with code pieces written by me as well. There is no particular structure nor do I pretend this to be a comprehensive guide. Also there are no explanations as the purpose is just to list important things. Moreover, this blog post is work-in-progress as I’m planning to add more things to it.
As always, opinions are mine.
Binary Search
A curated list of awesome Competitive Programming, Algorithm and Data Structure. Created with a view to connecting people to information, this list below contains a complete collection of all the fantastic resources I’ve collected over the course of my 11-year competitive programming career. The International Collegiate Programming Contest (ICPC). Of course, the book is also suitable for anybody else interested in competitive programming. It takes a long time to become a good competitive programmer, but it is also an opportunity to learn a lot. You can be sure that you will get a good general. Here you will get Kali Linux commands list (cheat sheet). Kali Linux is a Debian-based Linux distribution which was developed for penetration testing and security auditing. It provides various tools for testing security vulnerabilities. It is widely used by hackers for hacking purpose.
Breadth First Search
Depth First Search
Just replace the queue in BFS with stack and you are done:
Backtracking
Generally a recursive algorithm attempting candidates and either accepting them or rejecting (“backtracking”) based on some condition. Below is an example of permutations generation using backtracking:
Dynamic Programming
Technique to determine result using previous results. You should be able to reason about your solution recursively and be able to deduce dp[i] using dp[i-1]. Generally you would have something like this:
Dynamic Programming: Knapsack
Union Find
When you need to figure out if something belongs to the same set you can use the following:
Binary Tree Traversals
- Inorder: left-root-right
- Postorder: left-rigth-root
- Preorder: root-left-right
- Plus each one of them could have reverse for right and left
Below are recursive and iterative versions of inorder traversal
Segment Tree
Djikstra
Simplest way to remember Djikstra is to imagine it as BFS over PriorityQueue instead of normal Queue, where values in queue are best distances to nodes pending processing.
Multi-threading: Semaphore
Multi-threading: Runnable & synchronized
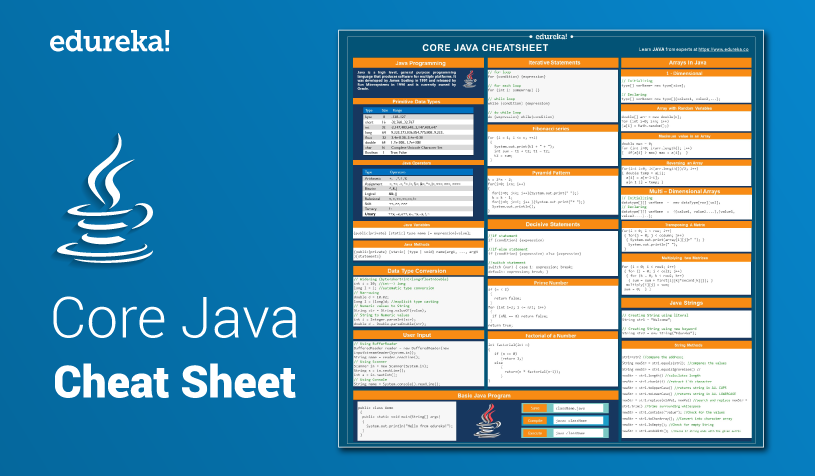
Strings: KMP
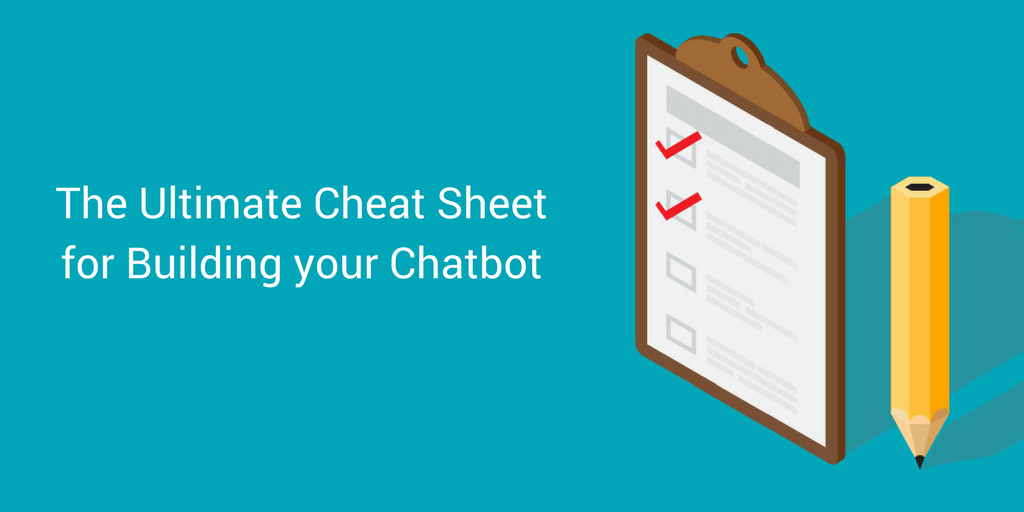
Hopefully no one asks you this one. It would be too much code here, but the idea is not that difficult: build LPS (example “AAABAAA” -> [0,1,2,0,1,2,3]); use LPS to skip when comparing as if you were doing it in brute-force.
Strings: Rolling Hash (Rabin-Karp)
Let’s imagine we have window of length k and string s, then the hash for the first window will be:
H(k) = s[0]*P^(k-1) + s[1]*P^(k-2) + … + s[k]*P^0
Moving the window by one, would be O(1) operation:
H(k+1) = (H(k) – s[0]*P^(k-1)) * P + s[k+1]*P^0
Here is some sample code:
Unit Testing
JUnit User guide: https://junit.org/junit5/docs/current/user-guide/
Data Structures
Many interview problems can be solved by using right data structure.
- hashmap
- stack
- queue
- priority queue
- trees
- linked list
- …
- Actually, better to go here: https://www.geeksforgeeks.org/data-structures/
System Design Topics
- requirements gathering
- capacity planning
- high level design
- components design
- database design
- API design
- queue
- map reduce
- long poll and push models
- load balancing
- partitioning
- replication
- consistency
- caching
- distributed caching
- networking
Some System Design Problems
- TinyURL
- Coming up with good encoding or better pre-building keys
- Handling collisions (if encoding or if different users have to have different key)
- Use distributed caching, CDN, LB
- Consider API throttling, eviction (maybe not), capacity
- HTTP 302
- Pastebin
- Separate data from metadata and store separately
- Otherwise similar to TinyURL
- Instagram
- Data partitioning is important here
- Pull vs push (via long polling) applied based on number of following
- Separate servers for viewing and posting for stability
- Generating feed of images requires sorting, consider timestamp for photoId
- Dropbox
- Client and Server
- Splitting files into chunks
- Saving metadata about chunks
- Local metadata database for the client
- Queues for load handling
- Data deduplication (user has same file in 100 places)
- Facebook Messenger
- poll vs push model with long polling
- push notifications
- Dedicate chat servers per user
- “seeing into the future” problem solving with seq number
- active status
- Twitter
- prebuild feed
- handle celebrities differently
- caching
- monitoring
- Youtube or Netflix
- Splitting files into chunks (time and quality) via MapReduce
- Distribution of content
- Thumbnail generator & distribution
- Store metadata separately (likes, views, comments, etc)
- CDN
- Typeahead Suggestion
- Trie
- For frequencies store them in nodes with suggestions
- Propagate suggestions independently from queries (mapreduce)
- Client implementation should delay request allowing for typing
- Client having local history
- API Rate Limiter
- DDoS
- Client identifier
- Sliding window with counters
- HTTP 429
- Web Crawler
- BFS
- Facebook’s Newsfeed
- Different feed components stored separately but joined
- Yelp
- Quad-Tree
- Uber
- Dynamic Quad-Tree
- HashMap
- Ticketmaster
- Relational DB design
- Concurrency handling
Resources
Well, internet is just full of all kinds of resources and blog posts on how to prepare for the technical interview plus there are numerous books and websites available. LeetCode is probably the most known of practicing websites. “Cracking The Coding Interview” is probably the most known book. I can also recommend “Guide to Competitive Programming”. As of system design, preparation resources are not so readily available. One good resource is “Grokking System Design Interview”, but it would be more valuable to practice designing large distributed systems. I also found the book “Designing Data-Intensive Applications” to be extremely helpful.
Asking for help
Competitive Programming Cheat Sheet Java
What are other “must know” algorithms and important system design topics to be listed in this post?
Bit Masking or Bit Manipulation is commonly used for various competitive programming questions, like, finding Unique number in an array of integers where each element occur twice or thrice, and we have print the unique number which appears only once.
There exists many other problems too, which can be easily solved by bit masking or bit manipulation.
Wpn firepotato games. Bit Manipulation basically means, we play with the inputs, or numbers bitwise.
We all know, a number can be represented in Binary format, where each bit is either 0 or 1, like, “2” can be represented as “10”, or “22” can be represented as “10110”.
We are going to discuss here, about the basic bits manipulations, We will discuss some more bit manipulations in further articles.
1. Getting a bit of a number
2. Setting a bit of a number

3. Clearing a bit of a number
Lets start with getting “i”th bit of a number, Suppose the given number is 8, and we have to extract its 2nd bit.
Binary representation of “8” : 1000, 0th bit = 0, 1st bit = 0, 2nd bit = 0, 3rd bit = 1.
N = 8, i = 2
1000 & 0100 <—— (AND with this mask) ——— 0000 <—— if this is “0” then “i”th bit is 0, if it is not zero, then “i”th bit is 1. ———
result = (N & mask) ? 1 : 0 = (N & (1<<i)) ? 1 : 0
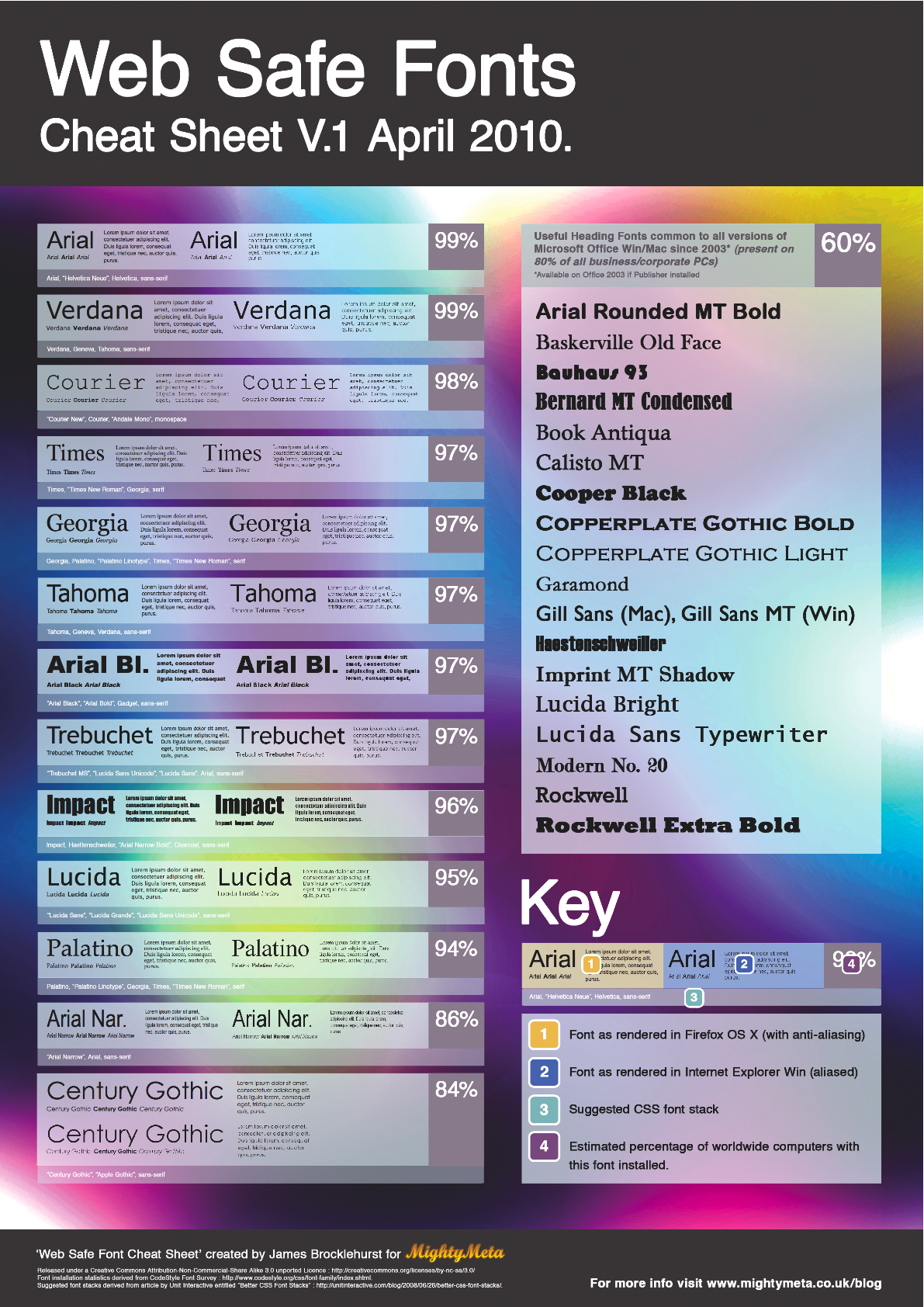
How to get this mask? simply, left shift “1”, “i” times, i.e., 1<<2 (i here is 2), we get, 100. Now, Do the AND operation of the number with mask. If the answer is non-zero then the ith bit is 1, else, ith bit 0.
Setting “i”th bit of a number
Competitive Programming Course
Suppose the given number is 11, and we have to set its 2nd bit.
N = 11, i = 3 11 in binary format : 1011, 0th bit = 1, 1st bit = 1, 2nd bit = 0, 3rd bit = 1.
1011 | 0100 <——- (OR with this mask) ———- 1111 <——- This is the result number after setting the “i”th bit. ———-
result = (N | mask) = (N | (1<<i))
How to get this mask? “1” left shift by “i”, i.e., 1<<2 = 100. Do the OR operatio of the number with this mask will get you the answer.
Clearing “i”th bit of a number
Competitive Programming Practice
N = 11, i = 1
1011 & 1101 <—– (AND with this mask) ——— 1001 ———
Mask is a number with all “1” bits except the “i”th bit : 1101, We may notice here that it is NOT of “0010”, How to get this second mask “0010”? Left shift “1” by “i”. Mathmr. regans educational website.
Competitive Programming Cheat Sheet
result = (N & (~(1<<i)))
