A short guide to all the exported functions in React Testing Library
- render
const {/* */} = render(Component)
returns:unmount
function to unmount the componentcontainer
reference to the DOM node where the component is mounted- all the queries from
DOM Testing Library
, bound to the document so thereis no need to pass a node as the first argument (usually, you can use thescreen
import instead)
- API Cheat Sheet – What is an API, How it Works, and How to Choose the Right API Testing Tools. Building an API is fun, right? Karate is an open-source tool for operations like API test-automation, performance-testing, UI automation into a.
- The one-page guide to RESTful API: usage, examples, links, snippets, and more.
Microsoft AZ-900 Cheat Sheet. The next important thing in this cheat sheet is to learn about the Microsoft AZ-900 exam. There has been a huge rise in the world of cloud computing from the past years. And, this is still increasing. With introducing courses like Microsoft AZ-900, Microsoft has made a clear pathway to start a career in cloud.
Queries#
Difference from DOM Testing Library
Dead zedgaming potatoes. The queries returned from render
in React Testing Library
are the same asDOM Testing Library
except they have the first argument bound to thedocument, so instead of getByText(node, 'text')
you do getByText('text')
See Which query should I use?
No Match | 1 Match | 1+ Match | Await? | |
---|---|---|---|---|
getBy | throw | return | throw | No |
findBy | throw | return | throw | Yes |
queryBy | null | return | throw | No |
getAllBy | throw | array | array | No |
findAllBy | throw | array | array | Yes |
queryAllBy | [] | array | array | No |
- ByLabelText find by label or aria-label text content
- getByLabelText
- queryByLabelText
- getAllByLabelText
- queryAllByLabelText
- findByLabelText
- findAllByLabelText
- ByPlaceholderText find by input placeholder value
- getByPlaceholderText
- queryByPlaceholderText
- getAllByPlaceholderText
- queryAllByPlaceholderText
- findByPlaceholderText
- findAllByPlaceholderText
- ByText find by element text content
- getByText
- queryByText
- getAllByText
- queryAllByText
- findByText
- findAllByText
- ByDisplayValue find by form element current value
- getByDisplayValue
- queryByDisplayValue
- getAllByDisplayValue
- queryAllByDisplayValue
- findByDisplayValue
- findAllByDisplayValue
- ByAltText find by img alt attribute
- getByAltText
- queryByAltText
- getAllByAltText
- queryAllByAltText
- findByAltText
- findAllByAltText
- ByTitle find by title attribute or svg title tag
- getByTitle
- queryByTitle
- getAllByTitle
- queryAllByTitle
- findByTitle
- findAllByTitle
- ByRole find by aria role
- getByRole
- queryByRole
- getAllByRole
- queryAllByRole
- findByRole
- findAllByRole
- ByTestId find by)
- configure change global options:
configure({testIdAttribute: 'my-data-test-id'})
- cleanup clears the DOM (use with
afterEach
to resetDOM between tests)
Text Match Options#
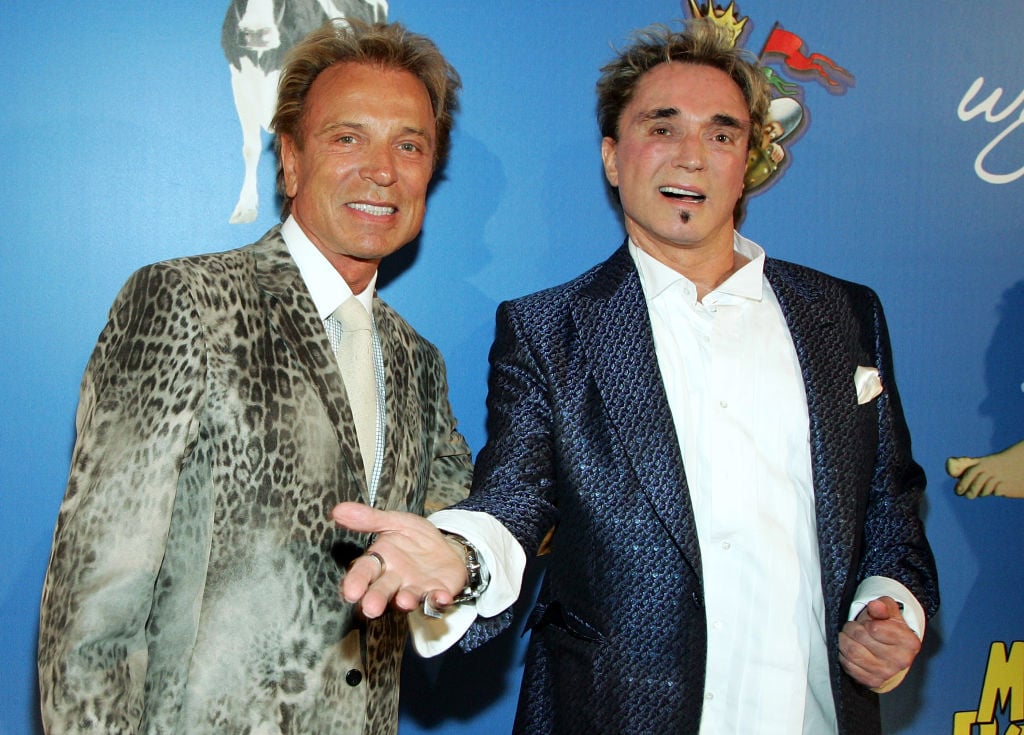
Given the following HTML:
Will find the div:
Api Testing Cheat Sheet Printable
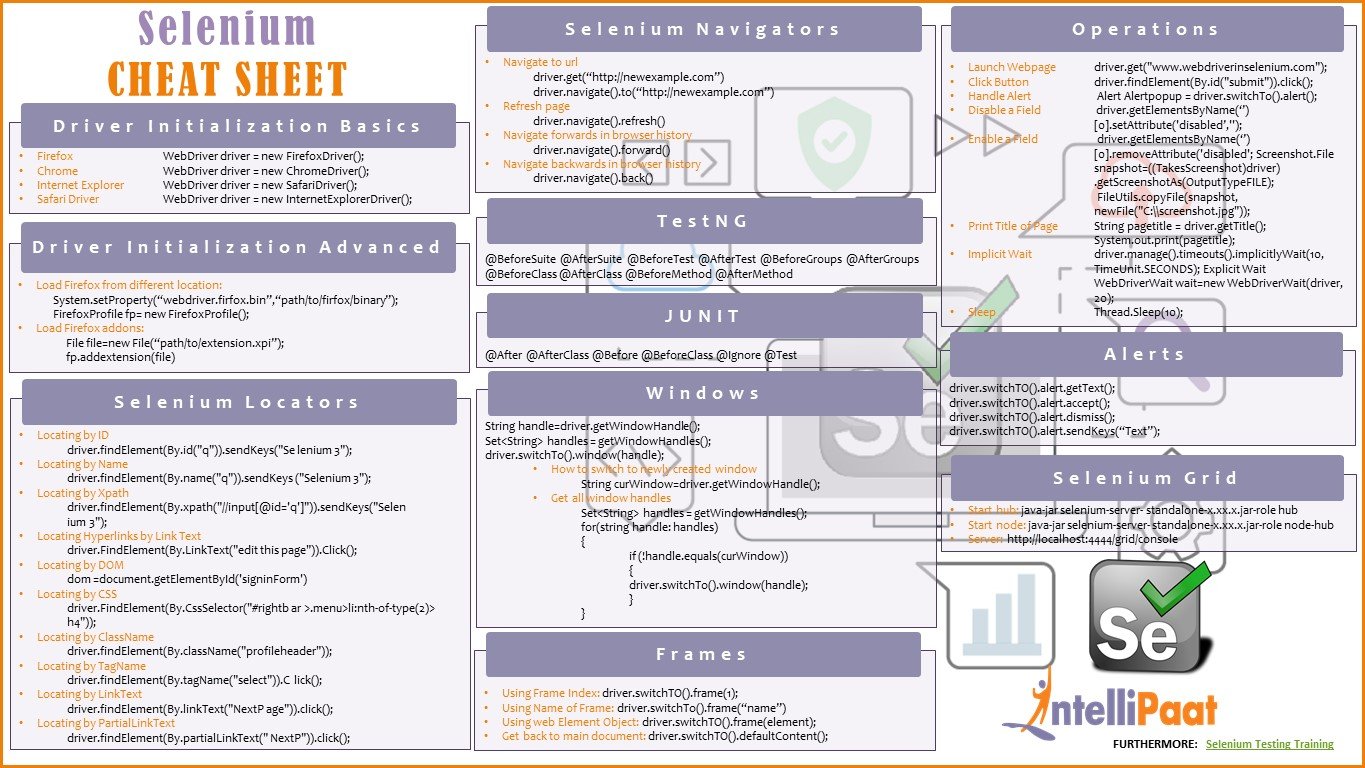
General
API
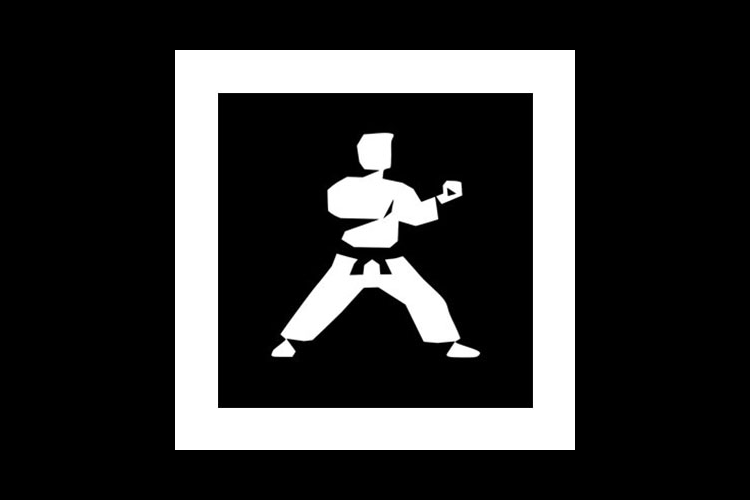
axios.request(config)axios.get(url[, config])axios.delete(url[, config])axios.head(url[, config])axios.options(url[, config])axios.post(url[, data[, config]])axios.put(url[, data[, config]])axios.patch(url[, data[, config]]) | |
axios.all(iterable)axios.spread(callback) | |
axios#create([config])axios#request(config)axios#get(url[, config])axios#delete(url[, config])axios#head(url[, config])axios#options(url[, config])axios#post(url[, data[, config]])axios#put(url[, data[, config]])axios#patch(url[, data[, config]]) |
Request Config
Response Schema
Config Defaults
Interceptors
Handling Errors
Api Testing Cheat Sheet Pdf
Cancellation
